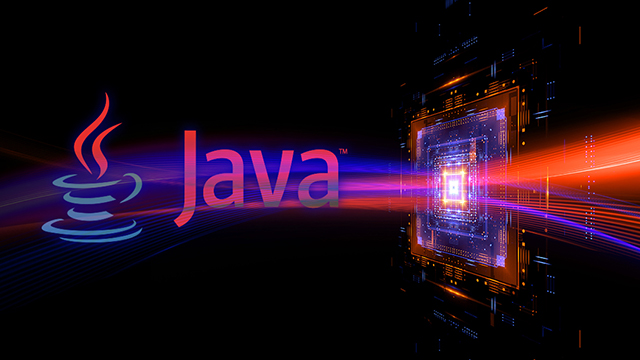
Java Programming OverviewThis course of study builds on the skills gained by students in Java Fundamentals or Java Foundations to help advance Java programming skills. Students will design object-oriented applications with Java and will create Java programs using hands-on, engaging activities.
Available Curriculum Languages:
- English (Student can ask queries in Hindi also for India)
Duration
- Recommended total course time: 40 hours
- Course time includes instruction, self-study/homework, practices and assignment.
Target Audiences Educators
- Technical, vocational, and 2- and 4-year college and university faculty members who teach computer programming or a related subject
- Secondary and vocational school teachers who teach computer programming
Students
- Students who wish to extend their programming experience in Java and develop more complex Java applications
- This course is a suitable foundational class for computer science majors and non-majors alike, and when taught in sequence with Java Fundamentals or Java Foundations, and may be used to prepare students for the AP Computer Science A exam.
Prerequisites
Required:
- Fundamental knowledge of object-oriented concepts, terminology, and syntax, and the steps required to create basic Java programs.
- A Preview into the IT World
- Software Development Life Cycle
- Project Module
Section 2: First Meeting with Java
- History of Java
- Java Technology
- Java Development Kit (JDK) and IDE Download
- Java Platform
- Interpretation Of Java Language by Computers
- Verification of JDK installation and running the first program
- Phases of a Java Program
- My First Java Program
Section3: Introduction to Object Oriented Programming
- Objects
- Software objects benefits
- Class
- Inheritance
- Interface
- Package
Section 4: Basics of Java Language
- Variables
- list of keywords
- kinds of variables
- Primitive Data Types
- Arrays
- Operators
- Types of operators
Section 5: Control Flow Statements
- Introduction
- if – then statement
- if – then – else Statement
- Switch Statement
- while and do-while Statements
- for Statement
- Break Statement
- Continue Statement
Section 6: Classes And Objects
- Java Escape Sequences
- Abstraction
- Encapsulation
- Relationships between classes
- Class Declaration
- Access Modifiers
- Member Variable Declaration
- Class Type Variable
- Method Declaration
- Constructors
- Types of constructors
- Constructor Overloading
- difference between constructor and method
- this and final keywords
- Enumeration
Section 7: Discussion on Interfaces And Inheritance
- Inheritance – Sub Classes, What exactly they are?
- Object Behaviour
- A Look At Polymorphism
- Overriding, an approach to override Methods
- The final keyword, to overcome overrides
- Class and Method Abstraction
- Interfaces – Implementation
- Interfaces – An alternative Data type
- Interface Editing
Section 8: The Reason To Have a Package
- Package Creation and Usage
- Create Your Own Package
- Naming a Package – Conventional Approach
- Accessing The Content Of A Package
- Accessing Package Member By Its Reference
- Importing Only Package Member
- Importing Entire Package
Section 9: Exceptions
- Exceptions – What exactly is it?
- Exception Classification
- Encountering An Exception
- Exception Handling
Section 10: Input Output Streams
- An Introduction
- Console Input
- Console Output
- Reading A File
- Writing To A File
- Creation Of Directories/Folders
- Reading Directories
Section 11: Meet the Threads
- An Introduction
- Lifecycle of A Thread
- Thread Priorities
- Creation Of Threads
- Thread Creation Using Runnable Interface
- Thread Creation Using Thread Class
- Thread Synchorization
Section 12: Applets
- Introducing Java Applets
- HTML – An Insight
- Applet Life Cycle
- First look at an Applet
- The Applet class in java.applet package
- Applet Implementation
- Embedding Applet in HTML
Section 13: Abstract Window Toolkit
- Introduction To Abstract Window Toolkit
- java.awt Package
- Creating Container
- Creating a Circle
- Button Creation
- Button Click Event
- Creating Checkbox
- Choice Option Implementation
Section 14: Swing
- Introduction to Swing
- Java Swing class hierarchy
- Swing With An Example
- JPanel and JFrame
- JWindow
- JTextField
- JButton
- JTextArea
Section 15: Collections
- Introduction
- The Collection Interfaces
- The Collection Classes
- The Collection Algorithms
- Iterator
- Comparator
- Client and Server Side Technologies
- What are Servlets?
- Servlets Architecture
- Installations and Configuration
- What is a Web Server
- HTTP (Hypertext Transport Protocol)
Section 2: Inside Servlets & Servlet Lifecycle
- Servlet Lifecycle Moments
- Servlets Packages.Interfaces,Classes
Section 3: The Servlet API
- Servlet Interface
- GenericServlet Class
- HttpServlet class
- Differennce between Get And Post
- HTTP Request Object
- HTTP Response object
- Send Redirect vs. Request Dispatching
Section 4: Other Web App Components
- Servlet Init Parameters
- Context Init Parameters
- Listeners
- Attributes
Section 5: Conversational State – Http Session
- Session ID
- Standard request methods
- Web Application Status Codes
- Advantages and Disadvantages of Hidden variable
- Cookies
- Session
Section 6: Basic JSP Learning
- What is JSP?
- Expressions
- JSP Lifecycle
- directives
- Replacing Scriptlets with EL
- MVC – The Model View Controller Pattern
Section 7: Filters
- Request Filters
- Response Filters
- Filter Chain
Section 8: JSTL
- Looping without scriptlet
- Core Tags
- Formatting tags
- SQL tags
- XML tags
- JSTL Functions
Section 9: Web App Development
- more about WAR files
- War File Structure